MQL4でEMA10がSMA20の上にあるか下にあるかをサブウインドウに表示させる簡単なインジケーターを作成しました。トレンド転換点を早い段階で捉えることができます。
説明
SMA20に対しEMA10が上にある場合は+1.0、下にある場合は-1.0をヒストグラムに表示させる単純なものです。プラスマイナスの反転した直後にエントリーすることができれば長い間トレンドに乗ることができます。
欠点としては、1分足では頻繁に反応し、特にレンジ相場では機能しにくいことです。順張り手法のためのツールですので、順張りが機能しない場面では同様に機能しにくいことになります。
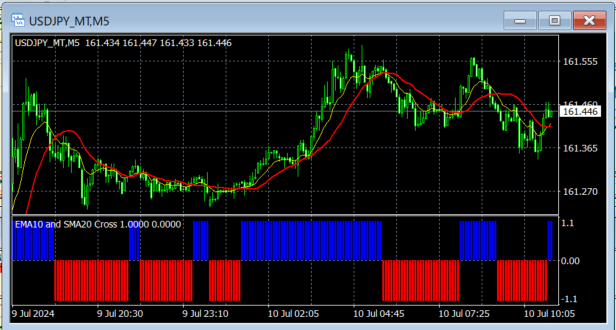
コード
//+------------------------------------------------------------------+
//| ema10Xsma20.mq4 |
//| Y.Urita |
//| https://rm-engineering.info |
//+------------------------------------------------------------------+
#property copyright "Y.Urita"
#property link "https://rm-engineering.info"
#property version "1.00"
#property strict
#property indicator_separate_window
#property indicator_buffers 2
// 色の入力パラメータ
input color colorPlus = clrBlue; // プラス側の色
input color colorMinus = clrRed; // マイナス側の色
// インジケーターバッファ
double BufferPlus[];
double BufferMinus[];
// 指定する時間足
ENUM_TIMEFRAMES timeFrames[] = {PERIOD_M1, PERIOD_M5, PERIOD_M15, PERIOD_H1, PERIOD_H4, PERIOD_D1};
// 現在のチャートの時間足を取得
ENUM_TIMEFRAMES currentTimeFrame;
//+------------------------------------------------------------------+
//| Custom indicator initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
// インジケーターバッファのセット
SetIndexBuffer(0, BufferPlus);
SetIndexBuffer(1, BufferMinus);
IndicatorShortName("EMA10 and SMA20 Cross");
// バッファの初期化
ArraySetAsSeries(BufferPlus, true);
ArraySetAsSeries(BufferMinus, true);
// 現在のチャートの時間足を取得
currentTimeFrame = (ENUM_TIMEFRAMES)Period(); // 明示的に型変換
// 色の設定
SetIndexStyle(0, DRAW_HISTOGRAM, STYLE_SOLID, 2, colorPlus);
SetIndexStyle(1, DRAW_HISTOGRAM, STYLE_SOLID, 2, colorMinus);
// バッファの描画開始位置を設定
SetIndexDrawBegin(0, 0);
SetIndexDrawBegin(1, 0);
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Custom indicator iteration function |
//+------------------------------------------------------------------+
int OnCalculate(const int rates_total,
const int prev_calculated,
const datetime &time[],
const double &open[],
const double &high[],
const double &low[],
const double &close[],
const long &tick_volume[],
const long &volume[],
const int &spread[])
{
// 必要なデータがあるか確認
if (rates_total < 20)
return(0);
int limit = rates_total - prev_calculated;
if (prev_calculated > 0)
limit++;
// バッファの初期化
for (int i = 0; i < limit; i++) {
BufferPlus[i] = 0;
BufferMinus[i] = 0;
}
for (int i = limit - 1; i >= 0; i--)
{
double ema10 = iMA(NULL, currentTimeFrame, 10, 0, MODE_EMA, PRICE_CLOSE, i);
double sma20 = iMA(NULL, currentTimeFrame, 20, 0, MODE_SMA, PRICE_CLOSE, i);
if(ema10 > sma20)
{
BufferPlus[i] = 1.0;
BufferMinus[i] = 0;
}
else if(ema10 < sma20)
{
BufferPlus[i] = 0;
BufferMinus[i] = -1.0;
}
}
return(rates_total);
}
コメント