MQL4を使った次の足までの時間を表示させるインジケーターです。
説明
画面右上に次の足までの残り時間が表示されます。
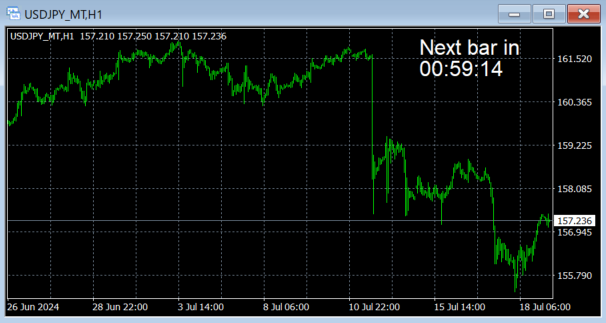
コード
//+------------------------------------------------------------------+
//| CountDownTimer.mq4 |
//| Y.Urita |
//| https://rm-engineering.info |
//+------------------------------------------------------------------+
#property copyright "Y.Urita"
#property link "https://rm-engineering.info"
#property version "1.00"
#property strict
#property indicator_chart_window
#property indicator_buffers 0
// 表示位置を定義します
input int countdownX = 200; // X座標
input int countdownY = 10; // Y座標
input color countdownColor = clrWhite; // テキストの色
input int fontSize = 16; // フォントサイズ
datetime lastBarTime; // 最後のバーの開始時間
//+------------------------------------------------------------------+
//| Custom indicator initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
// インジケータの初期化
lastBarTime = iTime(NULL, 0, 0); // 現在のバーの開始時間を取得
EventSetTimer(1); // 毎秒タイマーを設定
// 上段のラベルオブジェクトを作成
if (ObjectFind(0, "CountdownLabel") < 0)
{
ObjectCreate(0, "CountdownLabel", OBJ_LABEL, 0, 0, 0);
}
ObjectSetInteger(0, "CountdownLabel", OBJPROP_XDISTANCE, countdownX);
ObjectSetInteger(0, "CountdownLabel", OBJPROP_YDISTANCE, countdownY);
ObjectSetInteger(0, "CountdownLabel", OBJPROP_CORNER, CORNER_RIGHT_UPPER); // 右上に表示
ObjectSetInteger(0, "CountdownLabel", OBJPROP_FONTSIZE, fontSize);
ObjectSetInteger(0, "CountdownLabel", OBJPROP_COLOR, countdownColor);
ObjectSetInteger(0, "CountdownLabel", OBJPROP_BACK, true);
ObjectSetInteger(0, "CountdownLabel", OBJPROP_SELECTABLE, false);
ObjectSetInteger(0, "CountdownLabel", OBJPROP_HIDDEN, false);
ObjectSetInteger(0, "CountdownLabel", OBJPROP_ZORDER, 0);
ObjectSetString(0, "CountdownLabel", OBJPROP_TEXT, "Next bar in");
// 下段のカウントダウンタイマーオブジェクトを作成
if (ObjectFind(0, "CountdownTimer") < 0)
{
ObjectCreate(0, "CountdownTimer", OBJ_LABEL, 0, 0, 0);
}
ObjectSetInteger(0, "CountdownTimer", OBJPROP_XDISTANCE, countdownX);
ObjectSetInteger(0, "CountdownTimer", OBJPROP_YDISTANCE, countdownY + (fontSize *2)); // ラベルの下に配置
ObjectSetInteger(0, "CountdownTimer", OBJPROP_CORNER, CORNER_RIGHT_UPPER);
ObjectSetInteger(0, "CountdownTimer", OBJPROP_FONTSIZE, fontSize);
ObjectSetInteger(0, "CountdownTimer", OBJPROP_COLOR, countdownColor);
ObjectSetInteger(0, "CountdownTimer", OBJPROP_BACK, true);
ObjectSetInteger(0, "CountdownTimer", OBJPROP_SELECTABLE, false);
ObjectSetInteger(0, "CountdownTimer", OBJPROP_HIDDEN, false);
ObjectSetInteger(0, "CountdownTimer", OBJPROP_ZORDER, 0);
ObjectSetString(0, "CountdownTimer", OBJPROP_TEXT, "Initializing...");
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Custom indicator deinitialization function |
//+------------------------------------------------------------------+
void OnDeinit(const int reason)
{
EventKillTimer(); // タイマーを停止
ObjectDelete("CountdownLabel"); // ラベルオブジェクトを削除
ObjectDelete("CountdownTimer"); // インジケータのオブジェクトを削除
}
//+------------------------------------------------------------------+
//| Custom indicator calculation function |
//+------------------------------------------------------------------+
int OnCalculate(const int rates_total,
const int prev_calculated,
const datetime &time[],
const double &open[],
const double &high[],
const double &low[],
const double &close[],
const long &tick_volume[],
const long &volume[],
const int &spread[])
{
// OnCalculateでは特に計算しないが、必要に応じてここにコードを追加
return(rates_total);
}
//+------------------------------------------------------------------+
//| Timer function |
//+------------------------------------------------------------------+
void OnTimer()
{
RedrawCountdown();
}
//+------------------------------------------------------------------+
//| Custom function to redraw the countdown |
//+------------------------------------------------------------------+
void RedrawCountdown()
{
// 現在のバーの時間を取得
datetime currentBarTime = iTime(NULL, 0, 0);
// 新しいバーが始まった場合、lastBarTimeを更新
if (currentBarTime != lastBarTime)
{
lastBarTime = currentBarTime;
}
// 次のバーの開始時間を計算
datetime nextBarTime = lastBarTime + PeriodSeconds();
// 残り時間を計算
int remainingSeconds = (int)(nextBarTime - TimeCurrent());
// 時間、分、秒に分解
int hours = remainingSeconds / 3600;
int minutes = (remainingSeconds % 3600) / 60;
int seconds = remainingSeconds % 60;
// 残り時間を文字列にフォーマット
string countdownText = StringFormat("%02d:%02d:%02d", hours, minutes, seconds);
// チャートにテキストを表示
ObjectSetString(0, "CountdownTimer", OBJPROP_TEXT, countdownText);
ChartRedraw(); // チャートを再描画して更新
}
//+------------------------------------------------------------------+
コメント